React Native Troubleshooting Tips and Tricks
While building a React Native iOS and Android application, I've run into a number of trick, unintuitive issues. The following processes are how to solve them.
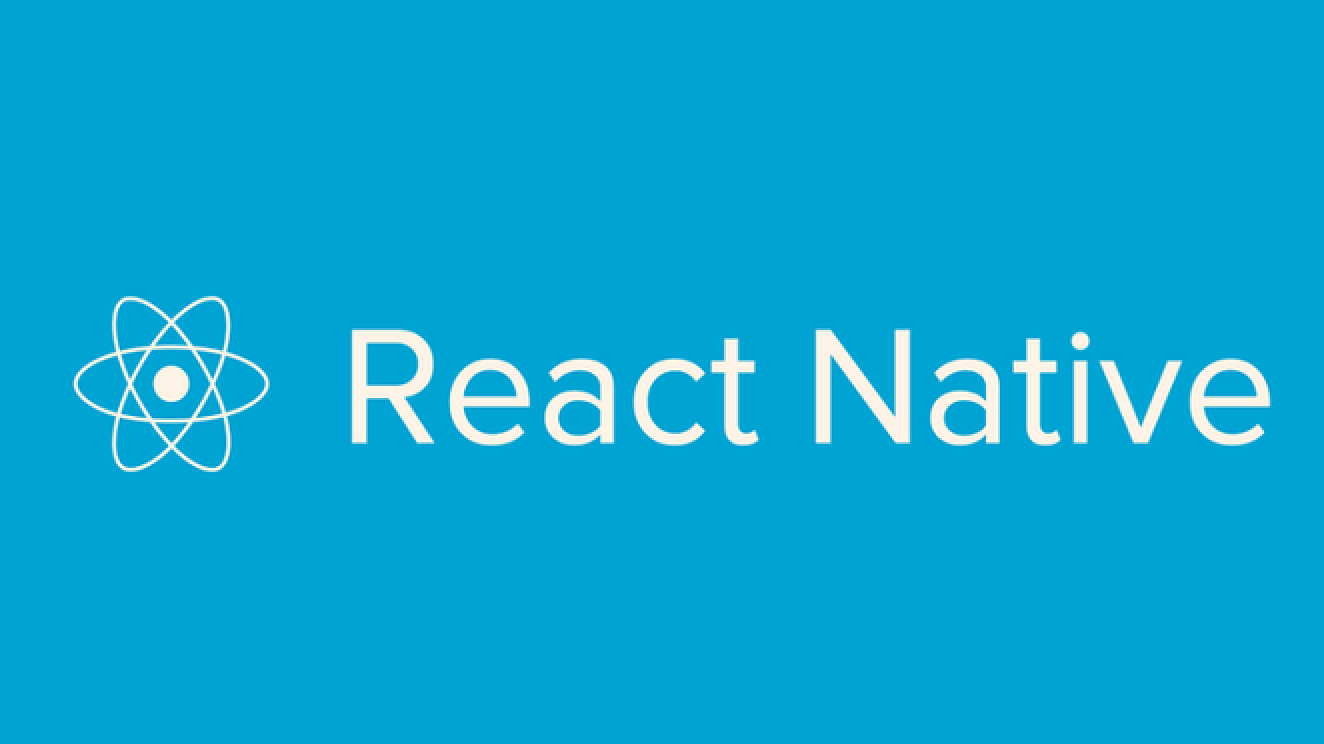
While building a React Native iOS and Android application, I've run into a number of tricky, unintuitive issues. The following processes and guide are how to solve weird issues.
React Native Debugging
General Process
- Checkout what the debugger has to say: http://localhost:8081/debugger-ui/
- Check language, package, framework and any other versions
- Update dependencies
- Kill any processes hanging around
- Restart your computer
- Bust a cache in that app
Specific Questions:
- Did you update packages or dependencies?
npm install && cd ios pod install
- Has NVM loaded the right Node version?
- Do you have all of the correct packages installed?
- Are you running the right version of XCode?
- Have you installed the XCode command-line tools?
- Are there any unlinked React Native packages? Link them.
- Are there processes hanging around? Kill them.
ps awwux | grep storybook
- Did you do something that requires a new build?
- Did configuration change or are you missing some secrets?
Cache Busting Cheatsheet
# React Native
rm -rf ~/.rncache
# Babel
rm ~/.babel.json
# iOS Build
rm -rf ios/build
# NPM - Cache and Clean Slate
npm cache clean --force # cache
rm -rf node_modules && npm install
# Watchman - clears watches/triggers
watchman watch-del-all
XCode Issues
1. "Could not parse the simulator list output"
dyld: Symbol not found: _SimDeviceBootKeyDisabledJobs
Referenced from: /Applications/Xcode.app/Contents/Developer/usr/bin/simctl
Expected in: /Library/Developer/PrivateFrameworks/CoreSimulator.framework/Versions/A/CoreSimulator
in /Applications/Xcode.app/Contents/Developer/usr/bin/simctl
Could not parse the simulator list output
Solution:
This one is pretty dumb. You just need to open and close the XCode app. This usually happens after an XCode upgrade.
2. pod install
fails because of glog
Installing glog (0.3.4)
<Thread:0x00007fd5a59c8060@/Users/stephen/.rvm/gems/ruby-2.5.1@brink-rn/gems/cocoapods-1.5.3/lib/cocoapods/executable.rb:166 run> terminated with exception (report_on_exception is true):
Solution:
(1) Double check you're using the correct version of the xcode CLI:
$ xcode-select -v
xcode-select version 2349.
(2) Double check your xcode-select
path. If it looks like the following, you'll want to relink it:
$ xcode-select -p
/Library/Developer/CommandLineTools
Relink to Xcode:
$ sudo xcode-select --switch /Applications/Xcode.app
pod install
should now succeed.
Source: https://github.com/facebook/react-native/issues/16279
3. "CocoaPods could not find compatible versions for pod"
This is probably because an NPM package got upgraded and the versions are out of sync. I would expect a manual pod install
to fail:
$ cd ios && pod install
Find the right package and run:
$ pod upgrade my-package-name
Everything should work now.
React Native Issues
Sources for assign MUST be an object.
TypeError: In this environment the sources for assign MUST be an object. This error is a performance optimization and not spec compliant.
This vague error means you've probably passing in the wrong value to a prop (e.g, boolean
when a prop is expecting an object
).
Local Assets aren't being rendered
After checking your paths and the React render chain to the point you think you're now crazy, take a look at the image docs. I can't find the original URL where I learned this but, by default, React Native won't render images larger than the device dimesions. Try this approach for large images:
...the packager will bundle and serve the image corresponding to device's screen density. For example, check@2x.png, will be used on an iPhone 7, whilecheck@3x.png will be used on an iPhone 7 Plus or a Nexus 5. If there is no image matching the screen density, the closest best option will be selected.
Flow Issues
If you run into weird flow issues, force a restart of the process or kill it entirely:
npx flow stop && npx flow start